Introduction
State management is a crucial aspect of building robust Flutter applications. It ensures that your app’s UI reflects the current state and allows you to manage changes effectively. This guide will cover the basics of state management in Flutter, including various approaches and best practices.
Table of Contents
- What is State Management?
- Types of State
- Built-in State Management
- Provider
- Riverpod
- Bloc
- Comparison of State Management Solutions
- Best Practices
What is State Management?
State management refers to the management of the state of one or more UI controls. It ensures that the UI is updated in response to state changes. The state in a Flutter application can include data such as user inputs, fetched data, or UI elements’ visibility.
Types of State
- Ephemeral State: Short-lived state that can be contained within a single widget. Examples include the current page of a
PageView
or a form field’s current input. - App State: State that affects multiple parts of the app or is required throughout the app’s lifecycle. Examples include user authentication status or theme settings.
Built-in State Management
Flutter provides built-in ways to manage state:
- StatefulWidget:
- Used for managing ephemeral state.
- Use
setState()
to trigger a rebuild when the state changes.
class MyStatefulWidget extends StatefulWidget { @override _MyStatefulWidgetState createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { int _counter = 0; void _incrementCounter() { setState(() { _counter++; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('State Management')), body: Center(child: Text('Counter: $_counter')), floatingActionButton: FloatingActionButton( onPressed: _incrementCounter, child: Icon(Icons.add), ), ); } }
Provider
Provider is a popular package for managing app state in Flutter. It uses the InheritedWidget
class internally and provides a simple, high-level interface for managing state.
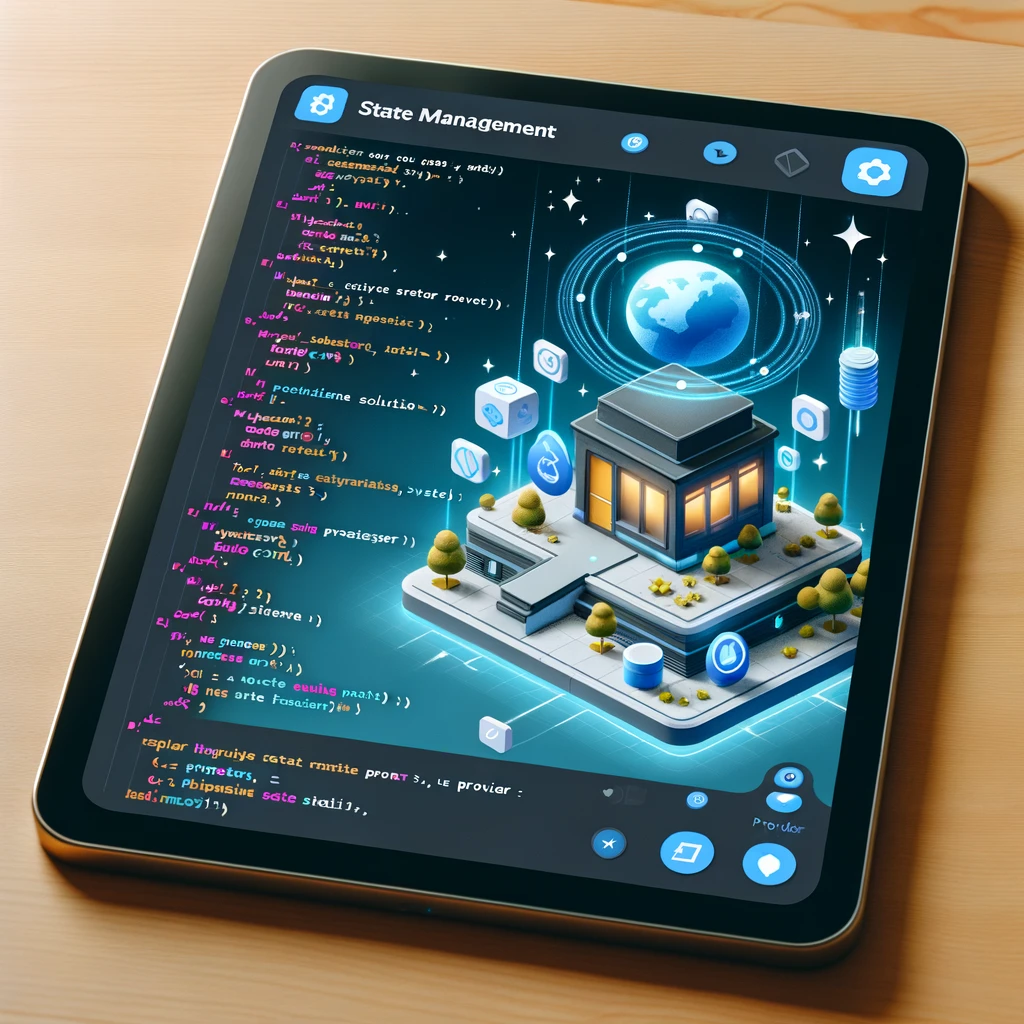
- Add Provider Dependency:yamlCopy code
dependencies: provider: ^5.0.0
- Create a ChangeNotifier Class:dartCopy code
class Counter extends ChangeNotifier { int _count = 0; int get count => _count; void increment() { _count++; notifyListeners(); } }
- Use Provider in the App:dartCopy code
void main() { runApp( ChangeNotifierProvider( create: (context) => Counter(), child: MyApp(), ), ); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: MyHomePage(), ); } } class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { final counter = Provider.of<Counter>(context); return Scaffold( appBar: AppBar(title: Text('Provider Example')), body: Center(child: Text('Counter: ${counter.count}')), floatingActionButton: FloatingActionButton( onPressed: counter.increment, child: Icon(Icons.add), ), ); } }
Riverpod
Riverpod is an improvement over Provider, offering better performance and a more robust API.
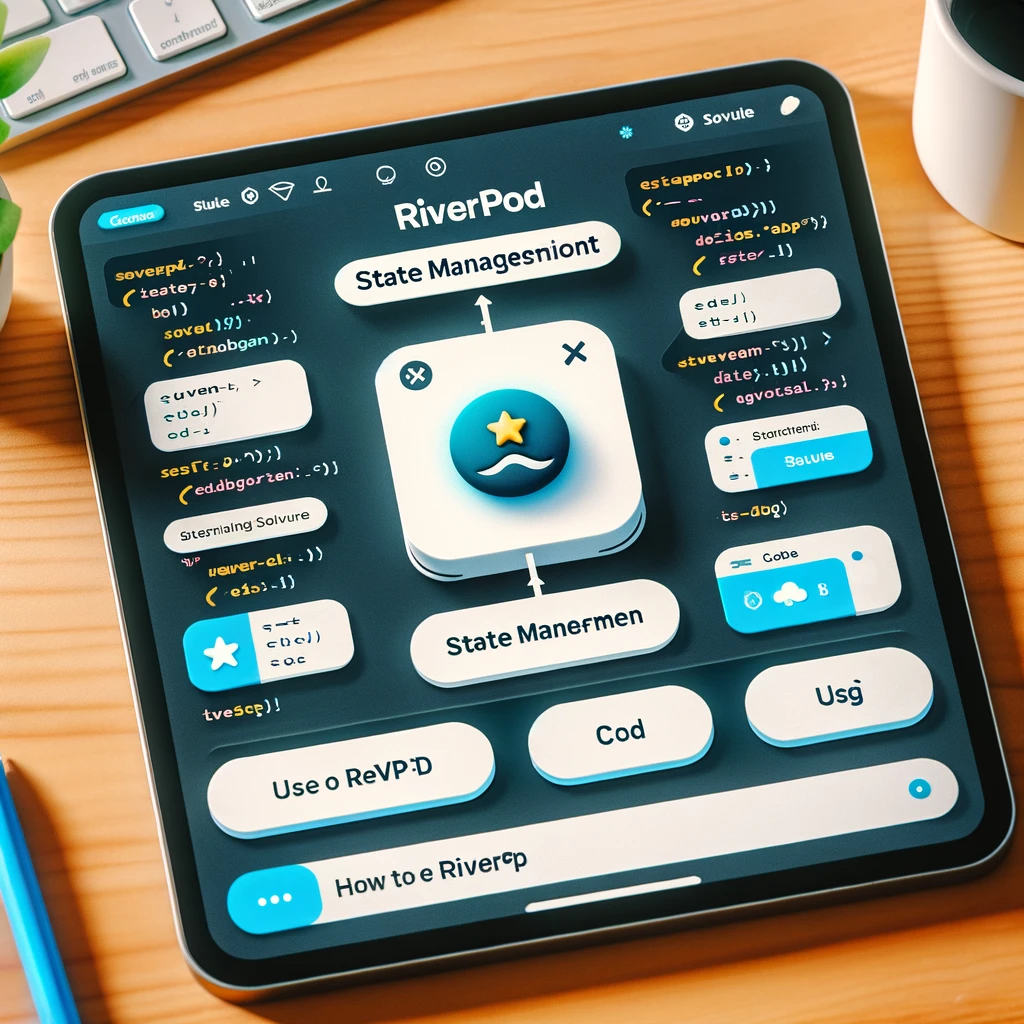
- Add Riverpod Dependency:yamlCopy code
dependencies: flutter_riverpod: ^0.14.0
- Create a Provider:dartCopy code
final counterProvider = StateNotifierProvider<CounterNotifier, int>((ref) => CounterNotifier()); class CounterNotifier extends StateNotifier<int> { CounterNotifier() : super(0); void increment() => state++; }
- Use Riverpod in the App:dartCopy code
void main() { runApp(ProviderScope(child: MyApp())); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: MyHomePage(), ); } } class MyHomePage extends ConsumerWidget { @override Widget build(BuildContext context, ScopedReader watch) { final count = watch(counterProvider); return Scaffold( appBar: AppBar(title: Text('Riverpod Example')), body: Center(child: Text('Counter: $count')), floatingActionButton: FloatingActionButton( onPressed: () => context.read(counterProvider.notifier).increment(), child: Icon(Icons.add), ), ); } }
Bloc
Bloc (Business Logic Component) is a powerful state management solution that separates presentation and business logic.
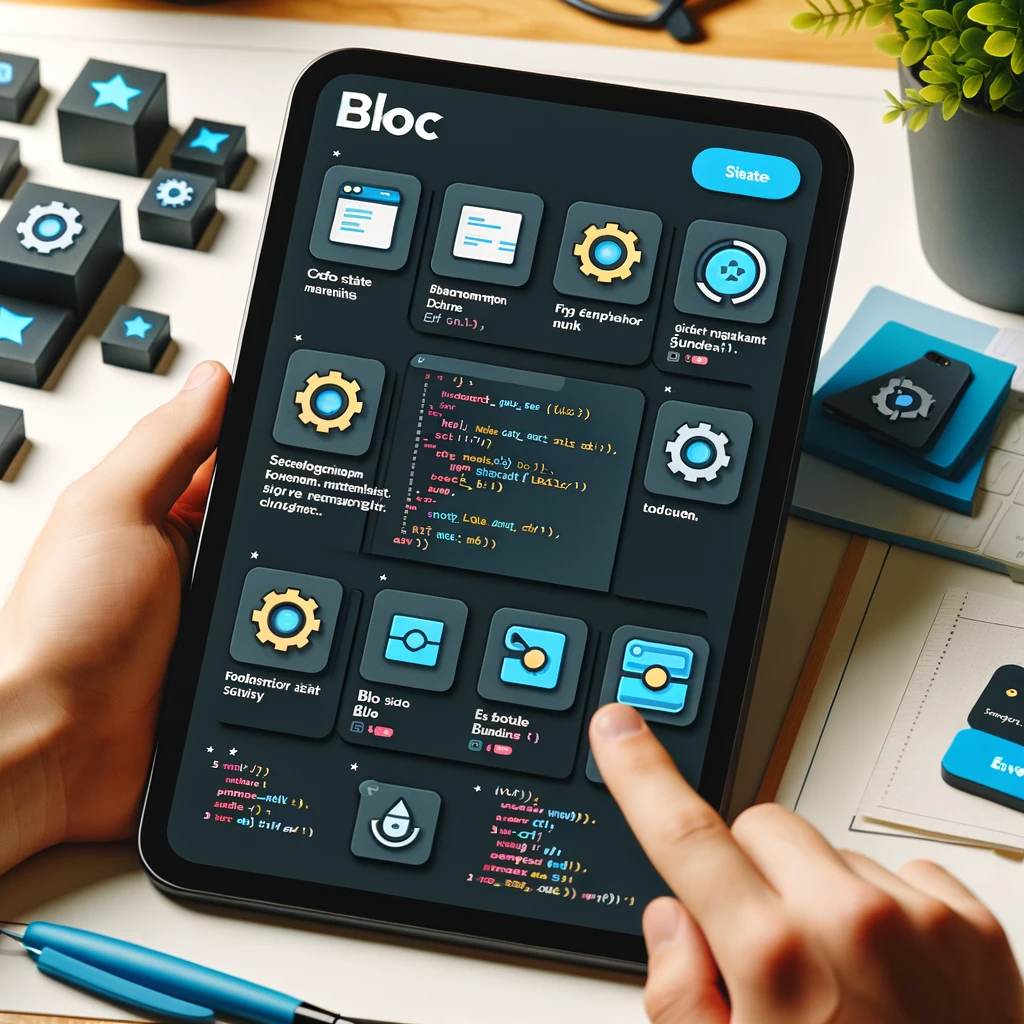
- Add Bloc Dependency:yamlCopy code
dependencies: flutter_bloc: ^7.0.0 bloc: ^7.0.0
- Create a Bloc:dartCopy code
class CounterCubit extends Cubit<int> { CounterCubit() : super(0); void increment() => emit(state + 1); }
- Use Bloc in the App:dartCopy code
void main() { runApp( BlocProvider( create: (context) => CounterCubit(), child: MyApp(), ), ); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: MyHomePage(), ); } } class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Bloc Example')), body: Center( child: BlocBuilder<CounterCubit, int>( builder: (context, count) { return Text('Counter: $count'); }, ), ), floatingActionButton: FloatingActionButton( onPressed: () => context.read<CounterCubit>().increment(), child: Icon(Icons.add), ), ); } }
Comparison of State Management Solutions
Solution | Description | Pros | Cons |
---|---|---|---|
Provider | Simple state management solution | Easy to use, widely adopted | Limited features |
Riverpod | Improved version of Provider | Better performance, more robust | Newer, less documentation |
Bloc | Powerful state management | Clear separation of logic/UI | More complex |
Best Practices
- Choose the Right Solution:
- Pick a state management solution that fits your app’s complexity and requirements.
- Keep State Logic Separate:
- Separate state management logic from UI code.
- Use Immutable State:
- Ensure that state objects are immutable to prevent unintended side effects.
Conclusion
Understanding state management in Flutter is crucial for building scalable and maintainable applications. By choosing the right state management solution and following best practices, you can ensure a smooth and efficient development process.